Type of constraints that reference
AnyParameterReference and ComponentParameterReference can constrain referencing types by specifying attributes.
Example script
|
|
Example of adding a script
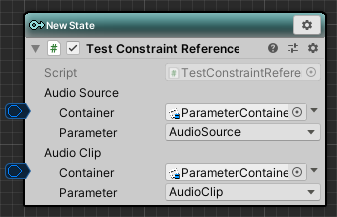
Example ParameterContainer
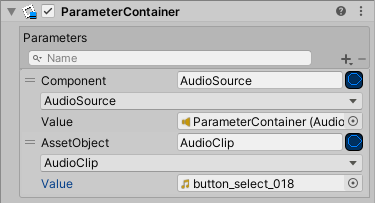
Examples of available attributes
Target type | Attributes |
---|---|
ComponentParameterReference | Class derived from ClassTypeConstraintAttribute SlotTypeAttribute It is also constrained to the Component class. |
AssetObjectParameterReference | Class derived from ClassTypeConstraintAttribute SlotTypeAttribute It is also constrained to the asset object type. |