Use of UniTask
If you have installed UniTask, the ToUniTask
method will be available.
Supported versions
UniTask Ver.2.0.32 or later
Preparation for use
When imported from Package Manager
It will be activated automatically.
No special preparation is required.
When importing unity package
Add ARBOR_SUPPORT_UNITASK
to Scripting Define Symbols in Player Settings.
Assembly definitions
If you are partitioning your assembly using Assembly definitions, you need to add a reference to Arbor.UniTask.asmdef.
If necessary, add a reference to “Assets/Plugins/Arbor/External/UniTask/Arbor.UniTask.asmdef” to the list of Assembly Definition References.
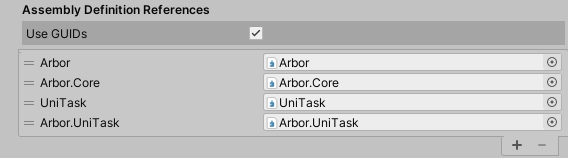
See Assembly definitions for details on how to configure.
See also here for the basic assembly definition of Arbor.
ToUniTask(YieldAwaitable)
Description
Convert YieldAwaitable to UniTask type.
You also need to add using Arbor.Threading.Tasks;
to use it.
Parameters
Parameter Name | Description |
---|---|
awaitable | YieldAwaitable to convert |
Returns
UniTask of the conversion result.
Example of use
This is an example of implementing async / await usage example in UniTask.
|
|