Type constraints of slots
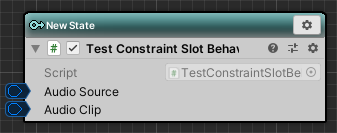
For some slots, you can use attributes to constrain the connectable types.
Example script
Create TestConstraintSlotBehaviour script file and write the following code.
|
|
Available Attributes
Class | Attribute |
---|---|
OutputSlotAny | SlotTypeAttribute |
InputSlotAny | Class derived from ClassTypeConstraintAttribute SlotTypeAttribute |
InputSlotComponent | Class derived from ClassTypeConstraintAttribute SlotTypeAttribute It is also constrained to the Component class. |
InputSlotUnityObject | Class derived from ClassTypeConstraintAttribute SlotTypeAttribute It is also constrained to the UnityEngine.Object class. |
FlexibleComponent | Class derived from ClassTypeConstraintAttribute SlotTypeAttribute It is also constrained to the Component class. |