Service
By creating a Service script, you can add your own update processing etc. by describing the processing you want to do.
Create Service script file
- Right click at the place you want to create from the Project window.
- From the right-click menu, select “Create> Arbor> Behavior tree> Service C# Script”.
- Enter the file name and confirm
Function to be called
When you add the created Service to the Composite node or Action node, each function of the script will be called.
- OnAwake
It is called the first time the node becomes active. - OnStart
It is called when the node becomes active. - OnUpdate
Called each time the node is active. For details about the execution timing, see Behavior tree's UpdateSettings.
Arbor Reference : BehaviourTree - OnAbort
Called when the node is interrupted by the Decorator. - OnEnd
Called when the node exits. - OnLateUpdate
It is called at the timing of BehaviourTree's LateUpdate().
Whether it is called every frame or not depends on BehaviourTree's Update Settings. - OnFixedUpdate
It is called at the timing of FixedUpdate() in BehaviourTree. - OnGraphPause
Called when a graph is paused while playing. - OnGraphResume
Called when a graph is resumed from pause. - OnGraphStop
Called when a graph stops playing. - MonoBehaviour message functions
For details, please see the MonoBehaviour Messages of Unity ScriptReference.
Call order
- If more than one service is added to the node, it is called from the top in order.
- You can also use the callback method of MonoBehaviour other than OnEnable and OnDisable, but please be aware that Start() will be called after the first OnUpdate().
Declaring variables
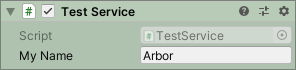
You can edit it in Arbor Editor by declaring a field with public or SerializedField attribute added to the created script.
|
|
Data flow
It is possible to input / output values by data flow.
For details, see “Scripting: Data flow”.
Additional explanation
- The file name and class name must be the same.
- If the file is placed under a special folder such as Editor folder, it will not be recognized.
- The AddComponentMenu("") that is automatically given is to prevent it from being displayed in Inspector's Add Component.
- We do not support the behavior of adding directly to GameObjects without going through the graph.
- The C# script needs to be compiled to reflect the changes.
If you have turned off Auto Refresh in Preferences, for example, you will need to manually do “Assets > Refresh” from the menu. - MonoBehaviour's enabled is used internally for execution control, so please use the alternative behaviourEnabled.